Python offers a myriad of functionalities, and one of them is the ability to interact with the file system on your computer. Today, we’re going to delve into a simple yet practical Python script that can calculate and list the sizes of all folders in a specified directory. This can be particularly useful for managing disk space, identifying large folders, and general system maintenance.
Understanding the Code
The script is divided into two main functions: get_folder_size and list_folder_sizes. Let’s break them down for a clearer understanding.
The get_folder_size Function
This function takes a file path as an input and calculates the total size of the folder. It walks through each file in the directory and its subdirectories using os.walk(). For each file, it checks if the file exists to avoid any errors related to file paths, and then adds its size to a total size counter. The size is calculated in bytes and then converted to gigabytes for easier comprehension.
The list_folder_sizes Function
The second function, list_folder_sizes, is used to display the sizes of all folders within a specified start path. It iterates through each directory in the given start path. For each directory, it calls the get_folder_size function and prints the size in a readable format (gigabytes).
Usage Example
To use this script, simply call the list_folder_sizes function with a directory path. For instance, list_folder_sizes(“C:\”) will list the sizes of all folders in your C drive.
Practical Applications
This script can be particularly handy for:
Identifying large folders that might be consuming unnecessary disk space.
System administrators for regular checks and maintenance.
Users seeking to clean up their drives and organize their files efficiently.
Conclusion
Python scripts like these demonstrate the power and simplicity of Python for everyday tasks. With just a few lines of code, you can gain valuable insights into your system’s file structure, helping you to manage your storage space more effectively. Whether you’re a seasoned programmer or a novice, Python’s ease of use and versatility make it an excellent choice for such tasks.
import os
def get_folder_size(path):
total_size = 0
for dirpath, dirnames, filenames in os.walk(path):
for f in filenames:
fp = os.path.join(dirpath, f)
if os.path.exists(fp):
total_size += os.path.getsize(fp)
return total_size
def list_folder_sizes(start_path):
for dirpath, dirnames, filenames in os.walk(start_path):
if dirpath == start_path:
for dirname in dirnames:
folder_path = os.path.join(dirpath, dirname)
size = get_folder_size(folder_path)
print(f”{folder_path} : {size / (102410241024)} GB”)
list_folder_sizes(“C:\”)
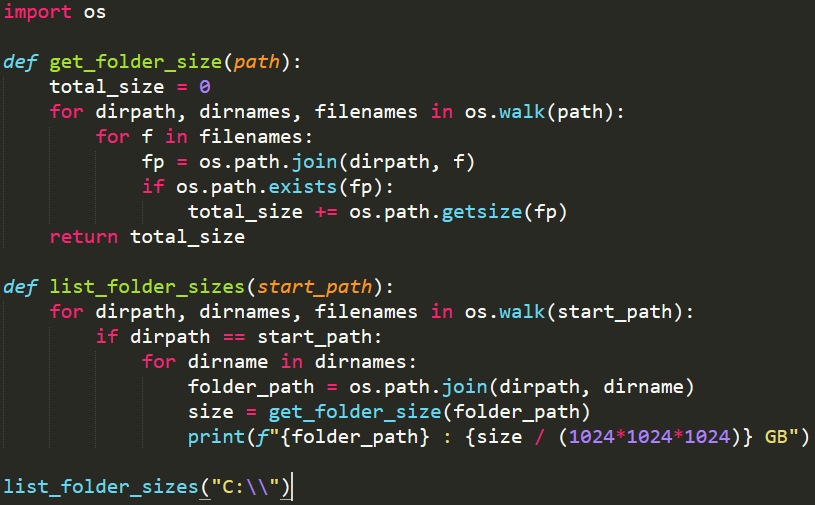